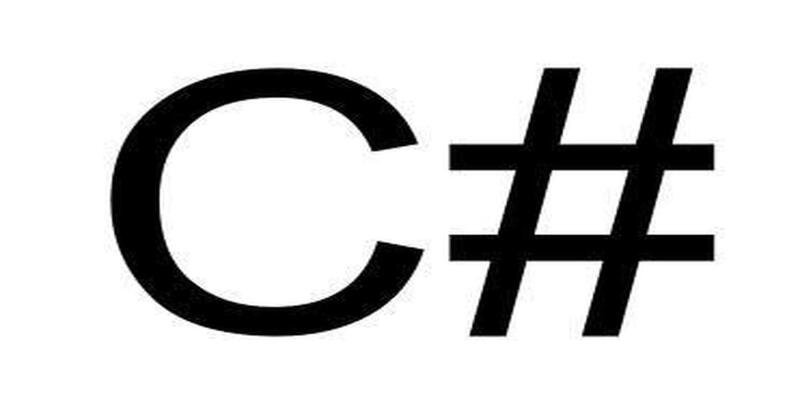
Hello, I'm starting with the same topic as my PHP For and While Loops tutorial because fundamentally everything is the same.
Loops are used to perform repetitive tasks. Initially, it may seem meaningless, but if you have a webpage with 100 members, instead of writing each name one by one, you can finish your work in three lines using a loop.
For Loop
The for loop revolves around the given increment value. It repeats the intermediate operations. Its syntax is as follows:
for( variable ; loop condition ; operation to be performed at each iteration)
variable: It allows us to create a variable at the beginning of the loop.
loop condition: This condition is actually an IF statement. If the question asked is true, it continues to revolve.
operation to be performed at each iteration: You don't always have to increase by one during the loop. You can change this operation and count by three or five.
Example:
int count; for ( count=1 ; count < 10 ; count++ ){ Console.WriteLine("I am currently at " + count); }
When you run this command, the following result will appear:
I am currently at 1
I am currently at 2
I am currently at 3
I am currently at 4
.... It continues like this 9 times. Since the given condition is count < 10, the iteration ends when count == 10.
While Loop
The while loop is a simpler version of the above. However, it is not only used for numbers. Since the while loop is dependent on a single condition, it should be used carefully.
Syntax:
While(Query) { operation to be performed if the query is true }
Example: Let's do the chick example.
int chick=0; while(chick>0){ Console.WriteLine("Hungry"); chick++; }
When you run this example, you will see Hungry written a million times on the screen. The reason is that chick is always greater than 0, and while loop asks the same question in each iteration, if the answer is Yes, it continues to iterate.
When we change the above example as follows, it will iterate only 10 times:
int chick=0; while (chick < 10){ Console.WriteLine("Hungry"); chick++; }
The most important part of this example is actually the chick++ line, which increases the value of chick by 1 at each iteration.
Türkçe: https://niyazi.net/tr/c-for-ve-while-donguleri
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment