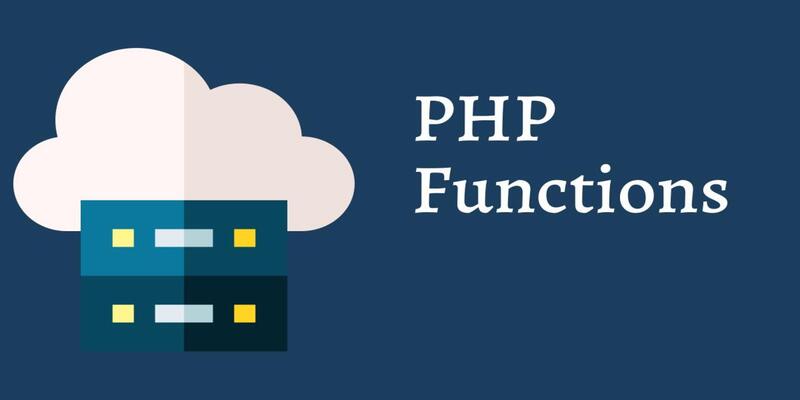
Functions provide us with processed information that is given, requested, or generated at that moment.
For example, we can create a table with the properties we want using a function, or we can create things like multiplication tables, etc.
The general usage is:
function FunctionName(){ echo "Niyazi Alpay"; }
This function, when called, will output Niyazi Alpay to the screen.
FunctionName();
is called like this.
Functions can perform different operations. This was a very simple operation; we just printed our name on the screen.
If we want to create a multiplication table, our function will be like this:
function CarpimTablosu(){
echo '<table border="1" align="center">';
for($i=0; $i<10; $i++){
echo '<tr>';
for($k=1; $k<=10; $k++){
echo '<td>'.($i+1).' x '.$k.' = '.($i+1)*$k.'</td>';
}
echo '</tr>';
}
echo '</table>';
}
CarpimTablosu();
Screen Output
Parameters in Functions
Parameters allow functions to receive values from outside and process that value to provide output.
Let's write a function that replaces the A characters in a given text with B. Here, we'll see another command: str_replace(); This is a function specific to the PHP library, and it's a parameterized function. Let me make the meaning of the parameter clearer with an example.
function Degis($yazi){
$yeni_yazi = str_replace('A','B',$yazi);
echo $yeni_yazi;
}
Now, the place we specified as $text when calling this function is the parameter. We defined this as a variable to be entered from the outside. When we call it like ReplaceA('NIYAZI ALPAY');, the screen output will be NIYBZI BLPBY.
str_replace(); function comes from string replace, it's a function that replaces a string value with another. As you can see in the example, this function has three parameters. The first one is the value we want to change, the second one is the new value, and the third one is the value affected by the change. Since we assigned this operation to a variable named $new_text, we echo it at the end to print it on the screen.
Now I'll show you a different usage.
function Degis($yazi){
$yeni_yazi = str_replace('A','B',$yazi);
return $yeni_yazi;
}
Here, we didn't use echo, instead, we used the return command. The difference is that it won't print anything to the screen when we call the function like ReplaceA('NIYAZI ALPAY');, but when we call echo ReplaceA('NIYAZI ALPAY');, we will see the output on the screen.
The difference is, in the first case, you're saying, "Bring me the book from the bag," and the function brings it to you, and in the second case, you're saying, "Bring me the bag, I'll take the book myself from it."
Now let's write a function to draw a table, let's enter the size of the table from the outside and print the cell number in each cell:
function TabloCiz($sart){
echo '<table border="1" align="center">';
for($i=0; $i<$sart; $i++){
echo '<tr>';
for($k=1; $k<=$sart; $k++){
echo '<td>'.($k+$i*$sart).'</td>';
}
echo '</tr>';
}
echo '</table>';
}
TabloCiz(10);
This time we set a parameter for this function. The parameter value is the size, so the function will create a table with as many cells as specified. For example, I entered 10 and created a 10x10 table, there are a total of 100 cells, and I printed the cell number in each cell.
I hope I've been able to explain the topic well. Good work.
Türkçe: https://niyazi.net/tr/php-de-fonksiyon-hazirlama-cagirma-ve-kullanma
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment