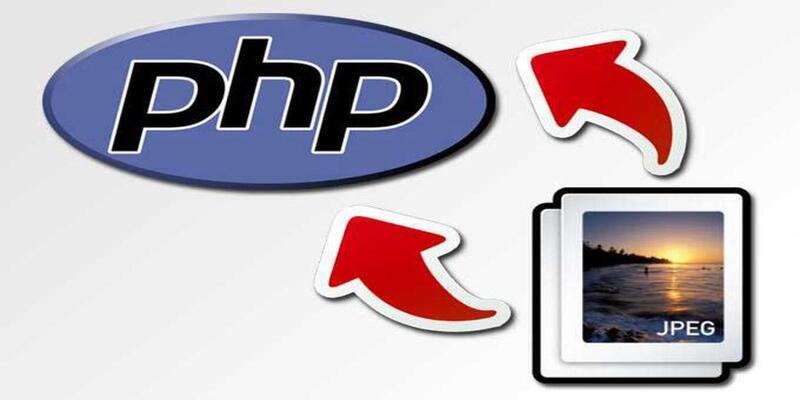
Hello, I'm going to show you the image resizing and uploading class I've prepared. In my previous post, I talked about object-oriented programming. The topic I'm going to explain now is a class for image resizing and uploading based on object-oriented programming. Without further ado, let's start explaining the class :)
<?php
class ResimIslem{
public function watermark($filigran, $source_file_path, $output_file_path )
{
list( $source_width, $source_height, $source_type ) = getimagesize( $source_file_path );
if ( $source_type === NULL )
{
return false;
}
switch ( $source_type )
{
case IMAGETYPE_GIF:
$source_gd_image = imagecreatefromgif( $source_file_path );
break;
case IMAGETYPE_JPEG:
$source_gd_image = imagecreatefromjpeg( $source_file_path );
break;
case IMAGETYPE_PNG:
$source_gd_image = imagecreatefrompng( $source_file_path );
break;
default:
return false;
}
$overlay_gd_image = imagecreatefrompng($filigran);
$overlay_width = imagesx( $overlay_gd_image );
$overlay_height = imagesy( $overlay_gd_image );
imagecopymerge(
$source_gd_image,
$overlay_gd_image,
$source_width - $overlay_width,
$source_height - $overlay_height,
0,
0,
$overlay_width,
$overlay_height,
60
);
imagejpeg( $source_gd_image, $output_file_path, 100 );
imagedestroy( $source_gd_image );
imagedestroy( $overlay_gd_image );
}
public function resim_boyutlandir($resim,$k_resim,$max_en=1920,$max_boy=1200){
// içeriği başlat..
$resimdosyasi= pathinfo($resim);
$uzanti=$resimdosyasi["extension"];
ob_start();
// ilk boyutlar..
$boyut = getimagesize($resim);
$en = $boyut[0];
$boy = $boyut[1];
// yeni boyutlar..
$x_oran = $max_en / $en;
$y_oran = $max_boy / $boy;
// boyutları orantıla..
if (($en <= $max_en) and ($boy <= $max_boy)){
$son_en = $en;
$son_boy = $boy;
}
elseif (($x_oran * $boy) < $max_boy){
$son_en = $max_en;
$son_boy = ceil($x_oran * $boy);
}
else{
$son_en = ceil($y_oran * $en);
$son_boy = $max_boy;
}
// uzantıya göre yeni resmi yarat..
switch($uzanti){
// jpg ve jpeg uzantılar için..
case 'jpg':
case 'jpeg':
// eski ve yeni resimler..
$eski = imagecreatefromjpeg($resim);
$yeni = imagecreatetruecolor($son_en,$son_boy);
// eski resmi yeniden oluştur..
imagecopyresampled($yeni,$eski,0,0,0,0,$son_en,$son_boy,$en,$boy);
// yeni resmi bas ve içeriği çek..
imagejpeg($yeni,null,85);
break;
// png uzantılar için..
case 'png':
$eski = imagecreatefrompng($resim);
$yeni = imagecreatetruecolor($son_en,$son_boy);
imagealphablending($yeni, false);
imagesavealpha($yeni, true);
$transparent = imagecolorallocatealpha($yeni, $son_en, $son_boy, $en, $boy);
imagefilledrectangle($yeni, 0, 0, $son_en, $son_boy, $transparent);
imagecopyresampled($yeni,$eski,0,0,0,0,$son_en,$son_boy,$en,$boy);
imagepng($yeni,null,-1);
break;
// gif uzantılar için..
case 'gif':
$eski = imagecreatefromgif($resim);
$yeni = imagecreatetruecolor($son_en,$son_boy);
imagealphablending($yeni, false);
imagesavealpha($yeni, true);
$transparent = imagecolorallocatealpha($yeni, $son_en, $son_boy, $en, $boy);
imagefilledrectangle($yeni, 0, 0, $son_en, $son_boy, $transparent);
imagecopyresampled($yeni,$eski,0,0,0,0,$son_en,$son_boy,$en,$boy);
imagegif($yeni,null);
break;
default:
$eski=null;
$yeni=null;
break;
}
$icerik = ob_get_contents();
// resimleri yoket ve içeriği çıkart..
ob_end_clean();
imagedestroy($eski);
imagedestroy($yeni);
$dosya = fopen($k_resim,"w+");
fwrite($dosya,$icerik);
fclose($dosya);
return $icerik;
}
private function adlandir($baslik){
$bul = array('Ç', 'Ş', 'Ğ', 'Ü', 'İ', 'Ö', 'ç', 'ş', 'ğ', 'ü', 'ö', 'ı', '-');
$yap = array('c', 's', 'g', 'u', 'i', 'o', 'c', 's', 'g', 'u', 'o', 'i', ' ');
$perma = strtolower(str_replace($bul, $yap, $baslik));
$perma = preg_replace("@[^A-Za-z0-9\-_]@i", ' ', $perma);
$perma = trim(preg_replace('/s+/',' ', $perma));
$perma = str_replace(' ', '-', $perma);
return $perma;
}
public function resim_upload($file_name,$file_tmp,$file_error,$file_path,$name,$en=1920,$boy=1200,$filigran=null){
$resimdosyasi= pathinfo($file_name);
$uzanti=$resimdosyasi["extension"];
$yeni_ad = $this->adlandir($name);
$yeni = $yeni_ad.'.'.$uzanti;
$max_en = $en;
$max_boy = $boy;
$b_resim = $file_path.'/orijinal-'.$yeni;
$k_resim = $file_path.'/'.$yeni;
if($uzanti=='jpg' or $uzanti=='jpeg' or $uzanti=='png' or $uzanti=='gif'){
if($file_error==0){
move_uploaded_file($file_tmp,$b_resim);
$this->resim_boyutlandir($b_resim,$k_resim,$max_en,$max_boy);
@unlink($b_resim);
///chmod($file_path, 755);
if($filigran!=null){
$this->watermark($filigran,$k_resim,$k_resim);
}
return $yeni;
}
else return $file_error;
}
else{
@unlink($k_resim);
return false;
}
}
}
?>
The first function in the class is used to find the extension of the uploaded file. The second function is for resizing the image. Typically, in image resizing, the transparent areas of PNG images turn black or get distorted in different ways. However, in this resizing function, the transparency of the image is preserved. The function takes the file information, maximum width and height as parameters, and processes the image accordingly.
The third function is used to rename the file, cleaning any Turkish characters from the filename. The last function is for uploading the image. It takes the file uploaded via POST, the folder or path where the file will be uploaded, the new name for the file, and the desired width and height as parameters. After uploading the image, this function finds the extension, renames the file, performs the upload process if the extension is a valid image file (jpg, jpeg, png, or gif), resizes the image again, and finally deletes the original file.
For those who don't want the image to be resized, the original image can be uploaded as it is. However, one advantage of resizing is that if the file is not really an image file, but is disguised as a JPEG file, it will be saved with a size of 0 bytes during resizing, effectively deleting its contents.
To use the class, save the provided code as "ResimIslem.php", include this file in the destination where you want to handle the uploaded images, and then use the following code:
$dosya = new ResimIslem();
$upload = $dosya->resim_upload(
$_FILES["formdan_gelen_resim"]["name"],
$_FILES["formdan_gelen_resim"]["tmp_name"],
$_FILES["formdan_gelen_resim"]["error"],
'yuklenecek/dizin',
'dosyanın adı',
1920,
1080,
'watermark-resmi'
);
echo $upload.' Dosyası başarıyla yüklendi.';
When a file is uploaded via POST, it has three different parameters. First, the file is temporarily stored in the server's temp directory and then transferred to the specified folder. The parameters obtained through POST include the file's name and temporary location (tmp). Additionally, the error parameter allows us to detect any potential errors that may occur during the upload process. By providing the directory, file name, and resizing parameters, we successfully upload the file. The function also returns the file name after the upload process, allowing us to save it in a database or display it on the screen using <img src="">
.
Furthermore, you can add a watermark to your image by specifying the path to the watermark image as 'watermark-resmi'.
In the next tutorial, I'll explain how to perform multiple image uploads using this class.
Türkçe: https://niyazi.net/tr/php-nesne-tabanli-programlama
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment