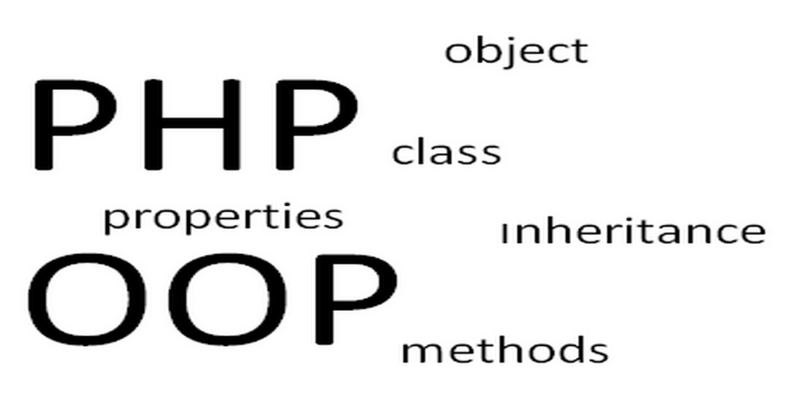
Object-oriented programming (OOP) is a technology used for software development. OOP facilitates component programming with its standards. C# is a language based on OOP. There are many objects available in C#, and programs are written using these objects. Additionally, we can write our own class.
OOP has three principles:
- Encapsulation
- Inheritance
- Polymorphism
Encapsulation: It refers to the information and operations about an object. These operations, called methods and properties, reveal the characteristics of the object, such as the color and size of a car.
Inheritance: It is the creation of an object based on another object, with the new object being influenced by the parent object. For example, a computer is composed of parts, and the parts are influenced by the full object of the computer.
Polymorphism: It is the use of a specific operation by multiple objects.
Object-Oriented Programming in PHP
To understand object-oriented programming in PHP, it is necessary to have a thorough knowledge of functions and variables, which are the basic building blocks of PHP.
We use the class command to create a class.
Let's create a file named class.php
<?php
class Computer{ }
?>
Our class is empty. After this, we cannot do anything other than variable declaration and function declaration here. The logic here is that we need to write a function specific to the class we are going to write and call the necessary function where necessary. It would not be appropriate to write a database class and then write a function to produce graphical statistics within it.
<?php
class Computer{
public $brand, price;
function defineBrand($newBrand){
$this->brand = $newBrand;
}
function showBrand(){
return $this->brand;
}
}
?>
We have created the functions defineBrand() and showBrand(). You can understand from the names what they do. Here, a special reference variable, $this->, is different from general usage. We use the $this-> expression to access a variable and access other functions of the current class.
With $this->brand = $newBrand, we assigned the value of $newBrand to the variable $brand, and with return $this->brand, we return the $brand variable.
Now, let's create an index.php file and include the class.php file.
<?php
include 'class.php';
?>
We only called the class.php file, but we are not using the computer object inside it yet. So let's continue.
<?php
include 'class.php';
$computer = new Computer();
?>
I assigned the computer object to the $computer variable. Think of this variable as a handle; we can hold and control the cup with it.
<?php
include 'class.php';
$computer = new Computer();
$computer->defineBrand('HP');
echo $computer->showBrand();
?>
We defined the brand with the defineBrand function and printed it to the screen with showBrand. We did all of these with the class and its methods.
In PHP, object-oriented programming is generally done like this.
This topic is not limited to these, it's a very deep topic, I just wrote it for introductory purposes.
Türkçe: https://niyazi.net/tr/php-nesne-tabanli-programlama
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment