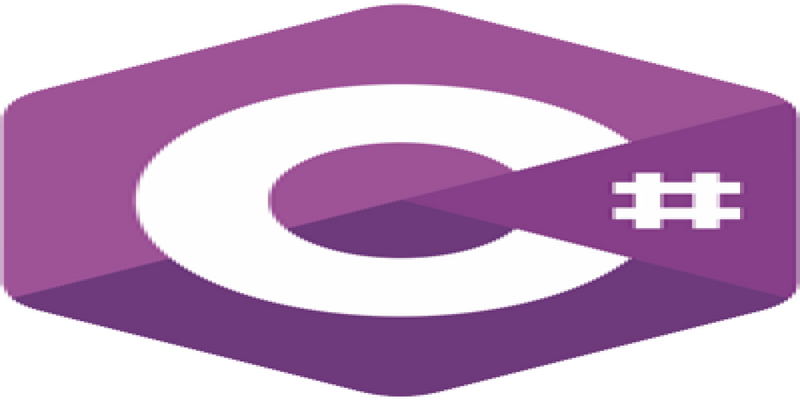
What is C#?
As you know, there are programs that we use to use our computers comfortably and programming languages are needed to make these programs. The most popular of these languages are Basic, C, C++, Pascal, Java and Assembler. A machine language is a set of commands defined by the hardware manufacturer to control the hardware. Some programming languages need compilers while others need interpreters, for example, to run a C++ program we need a C++ compiler, while for a CGI script written in Perl we need a command interpreter. Compilers translate the code into machine instructions before running the program, but interpreters interpret a group of codes line by line or block by block.
In fact, both compilers and command interpreters are nothing more than computer programs. In other words, C and C++ languages can be thought of as computer programs that wait for input and give output. These programs give source code as input and produce machine code as output.
A Brief Overview of C and C++ Languages
C is the most popular procedural programming language. It was developed by Dennis Ritchie, building upon the foundations of BCPL and B languages developed by Martin Richards and Ken Thompson.
The growth of the C language was greatly influenced by "The C Programming Language" book authored by Brian Kernighan and Dennis Ritchie. A significant milestone for the C language occurred in 1983 when the ANSI standards committee convened to establish standards for the language. This standardization process lasted for a total of 6 years. Another important development contributing to the current standards was the ANSI 99 standards.
C is a general-purpose language designed for programmers to develop various types of programs. With C, programmers can write programs for low-level systems as well as design high-level graphical user interfaces (GUIs). Additionally, developers can create their own libraries using C. Despite the passage of many years since its inception, the popularity of the C language has remained steadfast. Modern-day programmers continue to utilize source code written in C for various purposes.
Bjarne Stroustrup introduced the C++ language in the 1980s. C++ is considered a superset of C and is the most popular object-oriented programming language. Initially named "C with Classes," C++ is more efficient and powerful compared to C. Its most significant feature is its object-oriented nature, unlike C. Currently, the C++ language has been standardized by ANSI and ISO organizations. The latest version of these standards was released in 1997.
A Brief Overview of C# Language
C# is a powerful, modern, object-oriented, and type-safe programming language. It combines the strength of C++ with the ease of Visual Basic. Likely, the emergence of C# represents one of the most significant advancements in programming since the introduction of Java. Designed to harness the power of C++, the simplicity of Visual Basic, and the features of Java, C# is a versatile language.
It's worth noting that certain features found in Delphi and C++ Builder are now available in C#, although neither Delphi nor C++ Builder has ever achieved the popularity of Visual C++ or Visual Basic.
One of the major challenges for C and C++ programmers is the difficulty of rapid development, as they often deal with low-level concerns. With C#, however, this issue is alleviated. You can develop programs at both low and high levels in the same environment.
C# was developed by Anders Heljsberg, the leader of the team that created Turbo Pascal and Delphi, and Scott Wiltamuth, who worked on the Visual J++ team at Microsoft. It is positioned as the core and official language of the .NET platform.
The Common Language Runtime (CLR) in the .NET framework is similar to the Java Virtual Machine (JVM) in terms of garbage collection, reliability, and Just in Time (JIT) compilation. CLR serves as the execution environment for the .NET Framework, providing services and organizing code at runtime while adhering to ECMA standards.
In summary, using C# requires the CLR and .NET Framework class library. This means that C# is not simply C, C++, or Java; it is a new programming language that incorporates the best features of these languages. Ultimately, writing code in C# is advantageous, easy, and impressive.
Operators in C#
Aritmetik Operatörler
Operators Description
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus |
++ | Increment by One |
-- | Decrement by One |
Below is an example code demonstrating the usage of the operators listed in the table above. You can also download the code from here and try it out yourself.
public static void Main() { int toplam = 0, fark = 0, carpim = 0, kalan = 0; float bolum = 0; int sayi1 = 0, sayi2 = 0; Console.WriteLine("Sayı Biri Giriniz : " ); sayi1 = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Sayı İkiyi Giriniz : " ); sayi2 = Convert.ToInt32(Console.ReadLine()); toplam = sayi1 + sayi2; fark = sayi1 - sayi2; carpim = sayi1 * sayi2; kalan = sayi1 % sayi2; bolum = sayi1 / sayi2; Console.WriteLine("Girilen Sayılar: Sayı 1 = {0}, Sayı 2 = {1}",sayi1,sayi2); Console.WriteLine("Sayıların Toplamı = {0}",toplam); Console.WriteLine("Sayıların Farkı (sayi1 - sayi2) = {0}", fark); Console.WriteLine("Sayıların Çarpımı = {0}", carpim); Console.WriteLine("Sayıların kalan (sayi1 in sayi2 ye bölümğnden kalan)= {0}", kalan); Console.WriteLine("Sayıların Bölümünden (sayi1 / sayi2) Bölüm = {0}", bolum); sayi1++; sayi2--; Console.WriteLine("Sayi 1 in bir fazlası = {0}", sayi1); Console.WriteLine("Sayi 2 in bir eksiği = {0}", sayi2); }
If you examine the code above. Console.WriteLine("Description Text {0}", sayi1); you will see a structure like this. In string expressions, you can combine strings in this format instead of combining them as stringExpression1 + stringExpression2. The expression {0} means write the value of the first variable written after the comma here. If there is a second variable, we can put its value in another part by writing {1}. You need to pay attention that the numbers inside the {} expression should go in order. So 0, 1, 2,...
sayi1++ expression
sayi1 = sayi1 + 1;
has the same meaning. Also, if we want to add any number to a number, for example, let's add 5 to sayi1.
Instead of writing sayi1 = sayi1 +5;
sayi += 5;
is shorter and more accurate.
Using as Prefix and Postfix
The ++ and ? operators can be used as suffixes and suffixes. If we explain with examples;
sayi2 = 3;
sayi1 = ++sayi2;//sayi1 = 4, sayi2= 4.
sayi1 = --sayi2;//sayi1 = 2, sayi2= 2.
As seen in the example, if the operator is used as a prefix, the compiler first performs the task of the operator and then performs the assignment operation. Thus, in the expression sayi1 = +++sayi2, sayi2 is first increased by one and then the value of sayi2 is assigned to sayi1.
sayi2 = 3;
sayi1 = sayi2+++; //sayi1 = 3, sayi2= 4.
sayi1 = sayi2--; //sayi1 = 3, sayi2= 2.
When the operator is used as a postfix, the assignment operation is performed first and then the task of the operator is performed. In the operation numberi1 = numberi2++, first the value of numberi2 is assigned to numberi1, then numberi2 is increased by one.
Assignment Operators
Assignment Operators are used to assign values to variables.
Operator Description
= | Simple equalization |
+= | Add with the number on the right and then equalize the sum |
-= | Subtract the number on the right and then equalize the result |
*= | Multiply by the number on the right and then equalize the sum |
/= | Divide by the number on the right and then equalize the quotient |
%= | Find the remainder divided by the number on the right, then equal the remainder |
Relational (Comparison) Operators
Relational Operators are generally used for comparison in conditional statements. Relational operators used in C# are the following.
Operator Description
== | Equals |
!= | Not Equal |
> | Bigger |
< | Small |
>= | Great Equals |
<= | Small Equals |
Relational Operators always return a boolean value, either true or false. If we need to explain with an example;
int sayi1 = 6, sayi2 = 4;
sayi1 == sayi2 //false;
sayi1 != sayi2 //true;
sayi1 > sayi2 //true;
sayi1 < sayi2 //false;
sayi1 >= sayi2 //true;
sayi1 <= sayi2 //false;
Relational operators can only compare variables of compatible types. You cannot compare a boolean type with two integer type variables.
Logical and Bitwise Opeators
These operators are used to perform logical operations and bit-level operations.
Operator Description
& | Bit basis and operation |
| | Bit-wise or operation |
^ | Bit-wise xor operation |
! | Bit-by-bit note processing |
&& | Logical and operation |
|| | Logical or operation |
Bitwise operators process the value of a variable bit by bit. Logical operators, on the other hand, make comparisons within the scope of the concept of logic we know, such as whether this and that is true or whether this or that is true.
bool result = (i > 3 && j < 5) || (i < 7 && j > 3);
Logical operators;
True and True = True;
True and False = False;
False and True = False;
False and False = False;
True or True = True;
True or False = True;
False or True = True;
False or False = False;
In logical And operations, if any of the conditions is false, the result is false even if the other conditions subject to the and operation are true. In the Or operation, if any of the conditions is true, the result is true regardless of the other conditions.
C# Logical operators work with Short Circuit logic. In logical operations, conditions are checked from left to right. According to the information in the above paragraph; if an and operation is checked and one of the conditions is false, we can determine the result as false regardless of how many more and conditions are on the right. The C# compiler uses this method to interrupt the and operation the first time it sees false, and the or operation the first time it sees true, and returns the result. This allows our application to run faster when there are too many conditions in large applications.
Other Operators Used in C#
Operator Description
>> | Right shift by bit |
<< | Bit-wise left shift |
. | To access properties of objects |
[] | Accessing elements of arrays and collections by index number |
() | Cycle Operator. Used for type conversions. |
?: | Condition Operator. Short form of the if else condition. To be explained in the future |
Operator Work Priority
Not all operators have the same working priority. The order of precedence in multiplication, division, subtraction and addition, which is valid in mathematical rules, is also valid for operators. To determine the priorities, we need to write the operations in parentheses. For example
int i = 3 + 2 * 6; // first multiply 2 by 6, then add 3 to the product to get 15.
int j = (3 + 2) * 6; // first add 3 and 2, then multiply the sum by 6 to get 30.
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment