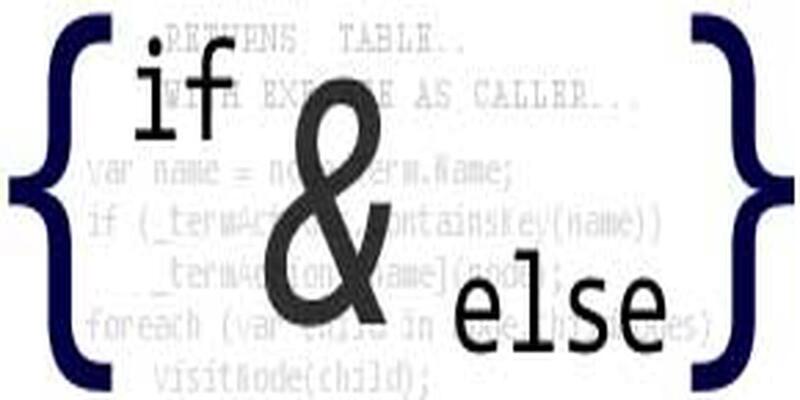
In C#, we declare variables according to their types, such as number type, text type, and so on.
We determined that "int a;" will be an integer type for the variable named 'a'.
We determined that "string b;" will be a string type for the variable named 'b'.
We defined that "double c;" will be a decimal number for the variable named 'c'.
Assignment of values to these variables can be done at any step of the program:
a=10;
b="Cryptograph";
c=5.5;
Variables are used for entering values from the outside, comparing these values, putting them into a loop, and doing other tasks. Any value can be entered from the outside, so it is a variable. To read a value entered from the outside, we use the Console.ReadLine() command. If we want to read a string type variable, we use b=Console.ReadLine();. But if we are going to read a number type variable, we should use a conversion function because the Console.ReadLine command reads as a string. a=Convert.ToInt32(Console.ReadLine()); this way, we read an int type variable, c = Convert.ToDouble(Console.ReadLine()); and with this command, we read a double type variable.
If-Else structure
It is used to compare a value entered from the outside or a value coming from a different source.
if (value to be compared) action to be taken in case of a match
else action to be taken if the comparison does not match
a=Convert.ToInt32(Console.ReadLine()); if(a==5) Console.WriteLine("Niyazi Alpay"); else Console.WriteLine("Wrong Number");
If the variable 'a' comes as 5, print Niyazi Alpay to the screen; otherwise, print Wrong Number.
If we want to perform multiple operations when the condition is met, we do it within {} parentheses.
a=Convert.ToInt32(Console.ReadLine()); if(a==5){ Console.WriteLine("Niyazi Alpay"); Console.WriteLine("Muhammad"); } else Console.WriteLine("Wrong Number");
We can also perform multiple comparisons.
a=Convert.ToInt32(Console.ReadLine()); if(a==5){ Console.WriteLine("Niyazi Alpay"); Console.WriteLine("Muhammad"); } else if(a==10) Console.WriteLine("Cryptograph"); else Console.WriteLine("Wrong Number");
The arithmetic characters we use when comparing are:
== if equal
> if greater
< if less
>= if greater than or equal to
<= if less than or equal to
=== if strictly equal
Türkçe: https://niyazi.net/tr/c-degisken-tanimlama-ve-if-else-yapisi
Muhammed Niyazi ALPAY - Cryptograph
Senior Software Developer & Senior Linux System Administrator
Meraklı
PHP MySQL MongoDB Python Linux Cyber Security
There are none comment